Vue.jsのプロジェクトにaxiosの共通モジュールをプラグインとして作成する
大体どんなプロジェクトでも使いそう。
まずvue-cliでVue.js 2系のデフォルトのプロジェクトを作ります。
そしてaxiosをプロジェクトにインストール。
$ npm install axios --save
import axios from "axios"
const AxiosPlugin = {}
AxiosPlugin.install = function (Vue) {
Vue.prototype.$axios = axios.create({
baseURL: "https://jsonplaceholder.typicode.com/",
// baseURL: process.env.VUE_APP_API_BASE_URL, .envファイルにURLを持たせた方がエンドポイントの切り替えに便利
headers: {
'Content-Type': 'application/json'
},
responseType: 'json',
timeout: 3000
})
// リクエストログ
Vue.prototype.$axios.interceptors.request.use(
function (config) {
console.info(config)
return config;
},
function (error) {
console.error(error)
return Promise.reject(error);
}
)
// レスポンスログ
Vue.prototype.$axios.interceptors.response.use(
function (response) {
console.info(response)
return response;
},
function (error) {
console.error(error)
return Promise.reject(error);
}
)
}
export default AxiosPlugin;
あとはこれをmain.jsで読み込むだけで「this.$axios」の形でaxiosのインスタンスが使えるようになります。
import Vue from 'vue'
import App from './App.vue'
// プラグインの読込
import AxiosPlugin from './plugins/axios';
Vue.use(AxiosPlugin)
Vue.config.productionTip = false
new Vue({
render: h => h(App),
}).$mount('#app')
<template>
<div id="app">
<input v-model="id" placeholder="id">
<button @click="getUser(id)">getUser</button>
<p>{{name}}</p>
</div>
</template>
<script>
export default {
data () {
return {
id: "",
name: "",
}
},
methods: {
getUser: function (id) {
this.$axios
.get("users/" + id)
.then(response => {
this.name = response.data.name
})
.catch(err => {
console.error(err)
})
}
}
}
</script>
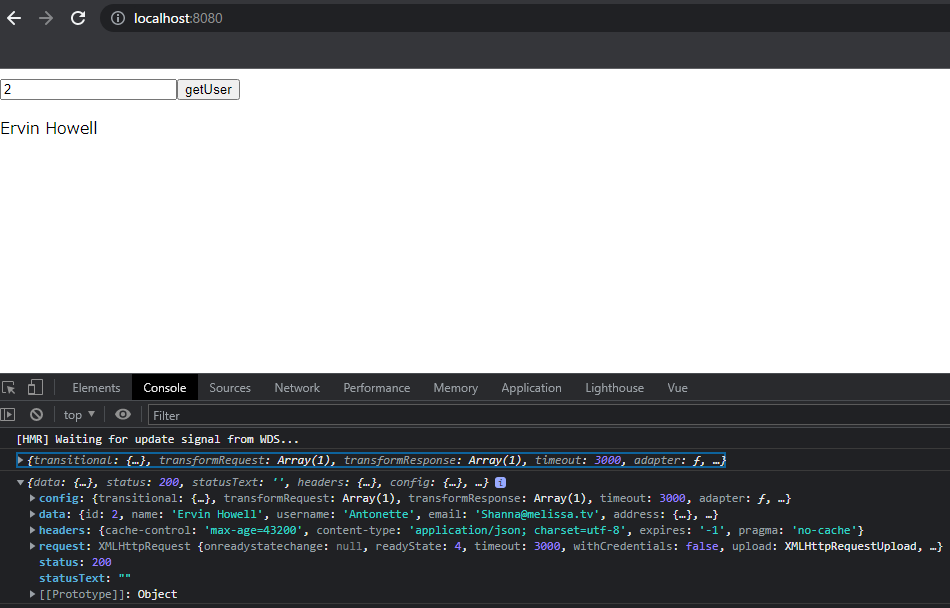
プラグインの設定を上書きする方法
例えばこのコンポーネントだけは別のAPIエンドポイントにアクセスするのでbaseURLを書き換えたいとか、デフォルトの設定を上書きたくなった場合。 this.$axios({
method: "GET",
baseURL: "https://jsonplaceholder.typicode.com/"
url: "users/" + id,
withCredentials: true
})
.then(response => {
this.name = response.data.name
})
.catch(err => {
console.error(err)
})
ディスカッション
コメント一覧
まだ、コメントがありません